Pure vs Impure Components in React
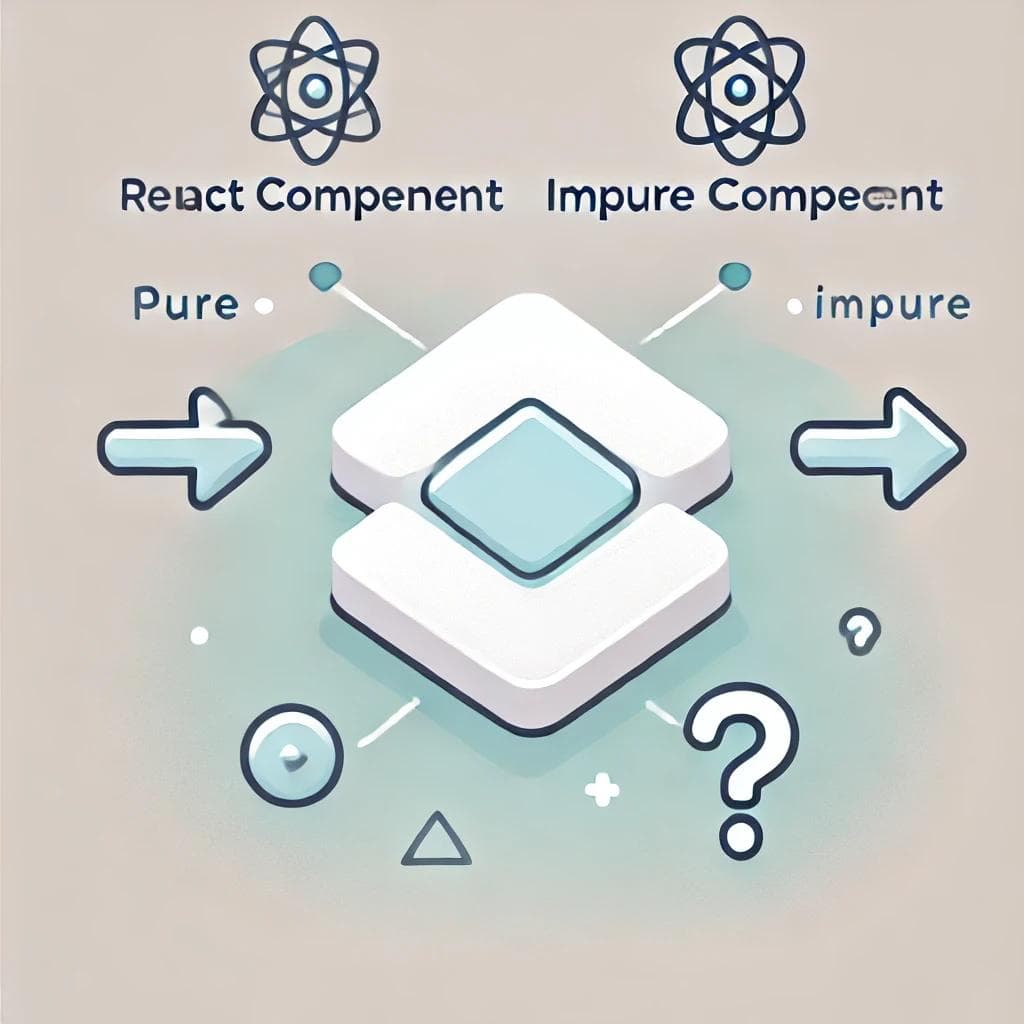
Understanding Pure vs Impure Components in React
In the world of React development, understanding the different types of components is crucial for writing clean, maintainable, and optimized code. In this blog post, we will explore two fundamental categories of components in React: Pure Components and Impure Components.
What are Components in React?
In React, components are the building blocks of the user interface. They are similar to JavaScript functions that accept inputs, known as props, and return a React element. This element describes how the UI should be rendered.
Components can be classified into two types: Pure Components and Impure Components.
1. Pure Component
A Pure Component in React is one that behaves predictably. It always returns the same output when provided the same input. Pure components do not rely on external state or side effects, making them easier to debug, test, and reason about.
Characteristics of Pure Components:
- Predictable output for the same props.
- Do not modify data outside their scope.
- Easily optimized by React for better performance.
Example of a Pure Component:
function PureButton(props) { const { onClick, children } = props; return <button onClick={onClick}>{children}</button>; }
In the above example, the PureButton
component will always render the same button with the same onClick
function and children
whenever it receives the same props.
2. Impure Component
An Impure Component, on the other hand, can have side effects, such as interacting with the DOM, modifying external state, or making network requests. Its output can change based on the current state of the component or the application, making it more complex to predict and debug.
Characteristics of Impure Components:
- Output may change even with the same input.
- Can interact with external state and modify data.
- Can cause unintended side effects.
Example of an Impure Component:
class ImpureCounter extends React.Component { state = { count: 0 }; handleClick = () => { this.setState({ count: this.state.count + 1 }); }; render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.handleClick}>Increment</button> </div> ); } }
In the ImpureCounter
example, the component’s output changes based on its state, which is modified when the button is clicked. This behavior makes it an impure component, as its output is not solely dependent on the props
.
Why Pure Components are Preferred
While impure components are necessary in some scenarios (e.g., handling user input, making API calls), it’s generally a good practice to write as many pure components as possible. Pure Components are easier to debug, more predictable, and can be optimized by React for better performance.
By leveraging Pure Components, you can ensure that your app runs efficiently, reducing unnecessary re-renders and improving user experience.