Dependencies vs. DevDependencies
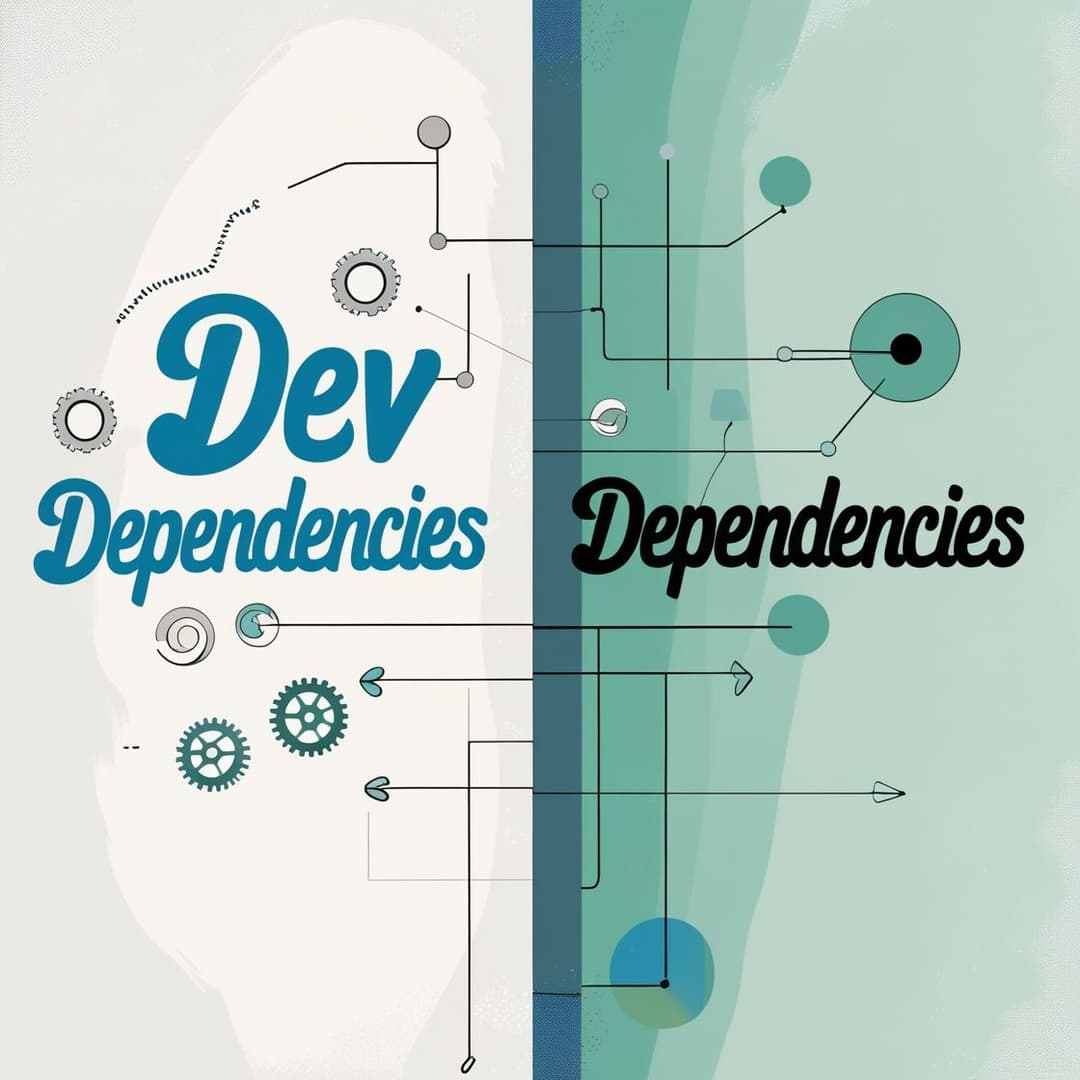
🚀 Are You Accidentally Slowing Down Your Production Apps?
Imagine this: your app is finally live, and users are excited to try it out. But there’s one problem—it’s painfully slow. 😟 What could have gone wrong?
If this sounds familiar, you’re not alone. One of the most common culprits behind sluggish production apps is unnecessary baggage: dev tools and dependencies that were never meant to make it to production. As developers, we often npm install
packages without a second thought. But are we truly paying attention to where these packages end up? 🤔
Let’s dive deeper into the issue and learn how to keep our production apps lean and efficient.
🔍 Dependencies vs. DevDependencies: Why It Matters
One of the most overlooked aspects of managing a project’s dependencies is understanding the distinction between dependencies and devDependencies. This distinction is crucial because it directly affects your app’s performance in production.
Dependencies 📦
Dependencies are the core building blocks of your app. These are the packages required for your app to function in production. Think of them as the essential ingredients in your recipe—they’re non-negotiable.
For example:
react
for building the user interfaceaxios
for making API callsexpress
for handling server-side requests
When you run the following command, the package is added to the dependencies
section in your package.json
file:
npm install react
DevDependencies 🔧
On the other hand, devDependencies are like the tools in your kitchen. They’re essential during development but completely unnecessary for the production environment. These include tools for building, testing, and debugging your app.
For example:
eslint
for linting your codejest
for testingwebpack
for bundling your application
When you run the following command, the package is added to the devDependencies
section in your package.json
:
npm install eslint --save-dev
💥 The Hidden Cost of Mistakes
So, what happens when you accidentally include devDependencies in your production build? Here are the consequences:
-
📂 Inflated Bundle Size
Including unnecessary tools in production increases your app's bundle size, which means users need to download more data, leading to slower load times. -
🐢 Reduced Performance
The extra weight impacts your app’s performance, making it feel sluggish and unresponsive. This can frustrate users and increase bounce rates. -
🔓 Increased Security Risks
Dev tools aren’t designed to be exposed in production. Including them can inadvertently introduce vulnerabilities, putting your app and users at risk.
✅ Best Practices to Keep Your App Lean
Now that we understand the problem, let’s look at actionable ways to prevent devDependencies from sneaking into your production environment.
1. Use the Right Flag 🏷️
When installing packages, always specify whether they’re for development or production. Use the --save-dev
flag for dev tools:
npm install eslint --save-dev
This ensures the package is categorized correctly in your package.json
.
2. Audit Dependencies Regularly 🕵️♂️
Over time, your project may accumulate unused or outdated packages. Regular audits can help you identify and remove unnecessary dependencies.
- Use
npm audit
to detect vulnerabilities. - Use
yarn why <package>
to understand why a package is in your project.
3. Prune Before Deploying ✂️
Before deploying your app, ensure that devDependencies are excluded from the production build. The npm prune
command removes unnecessary packages:
npm prune --production
4. Monitor Build Size 📊
Keep an eye on your app’s bundle size using tools like:
- Webpack Bundle Analyzer to visualize the size of your bundles.
- The following command to calculate the size of
node_modules
:npm install --production && du -sh node_modules
5. Educate Your Team 👩💻
A well-informed team is your best defense against mistakes. Take the time to educate your team about the importance of managing dependencies properly. Encourage practices like code reviews to catch potential issues early.
🛠️ Real-Life Example: Fixing the Problem
Imagine you’ve accidentally included webpack
(a dev tool) in your production build. Here's how you can fix it:
-
Identify the problem:
Usenpm ls webpack
to confirm where the package is being used. -
Move it to
devDependencies
:npm install webpack --save-dev
-
Prune the project:
npm prune --production
This process ensures your production environment remains lean and optimized.
🔧 Quick Tip for a Faster App
💡 Want a leaner, faster app? Start by trimming the fat! Pay close attention to your dependencies, audit regularly, and prune before deploying. Small changes can make a big difference.